SQL and Java are two different computer languages. SQL is used for working with databases, like asking questions or changing information. It’s simpler to learn because it has fewer rules for writing. Java, on the other hand, is more complicated because it’s used for many things, like making computer programs or websites.
Learning Java means understanding more about how computers work and how to solve problems with them. Both languages have their challenges, but starting with SQL might be easier for beginners.
It’s like learning to ask questions and make changes in a special way, while Java is like learning to build things from scratch with lots of tools and parts.
What are the basic concepts and syntax of SQL and Java?
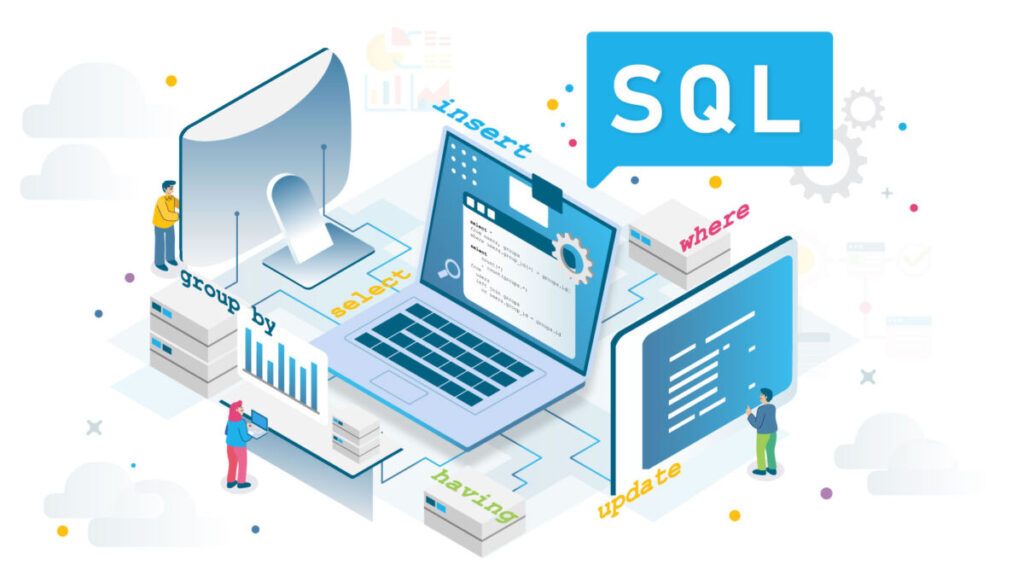
SQL (Structured Query Language) and Java are two distinct programming languages, each serving different purposes. Here’s a brief overview of their basic concepts and syntax:
SQL
SQL is a domain-specific language used for managing and manipulating relational databases. Its primary functions include querying, inserting, updating, and deleting data from databases.
Basic Concepts
- Database: A structured collection of data organized for efficient retrieval.
- Table: A collection of related data organized into rows and columns.
- Query: A request for data or information from a database.
- Data Manipulation Language (DML): SQL commands used for managing data in a database, such as SELECT, INSERT, UPDATE, DELETE.
- Data Definition Language (DDL): SQL commands used for defining and modifying database structure, such as CREATE, ALTER, DROP.
- Constraint: Rules defined on columns to enforce data integrity, such as NOT NULL, UNIQUE, FOREIGN KEY.
Syntax Example
— Creating a table
CREATE TABLE Students (
id INT PRIMARY KEY,
name VARCHAR(50),
age INT
);
— Inserting data
INSERT INTO Students (id, name, age) VALUES (1, ‘John Doe’, 25);
— Selecting data
SELECT * FROM Students;
— Updating data
UPDATE Students SET age = 26 WHERE id = 1;
— Deleting data
DELETE FROM Students WHERE id = 1;
Java
Java is a general-purpose, object-oriented programming language used for building various types of applications, including desktop, web, and mobile applications.
Basic Concepts
- Class: Blueprint for creating objects. It defines the properties (fields) and behaviors (methods) of objects.
- Object: An instance of a class that contains data and methods.
- Inheritance: Mechanism by which one class inherits properties and behaviors from another class.
- Polymorphism: Ability of objects to take on different forms depending on the context.
- Encapsulation: Bundling of data and methods that operate on the data into a single unit (class), restricting access to the inner workings of the object.
- Syntax: Java syntax is based on C and C++ syntax, with its own unique features.
Syntax Example
// Defining a class
public class Person {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter and Setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
// Creating an object and using it
public class Main {
public static void main(String[] args) {
// Creating an object of the Person class
Person person = new Person(“John Doe”, 25);
// Accessing object properties and methods
System.out.println(“Name: ” + person.getName());
System.out.println(“Age: ” + person.getAge());
}
}
How does the complexity of Java compare to SQL?
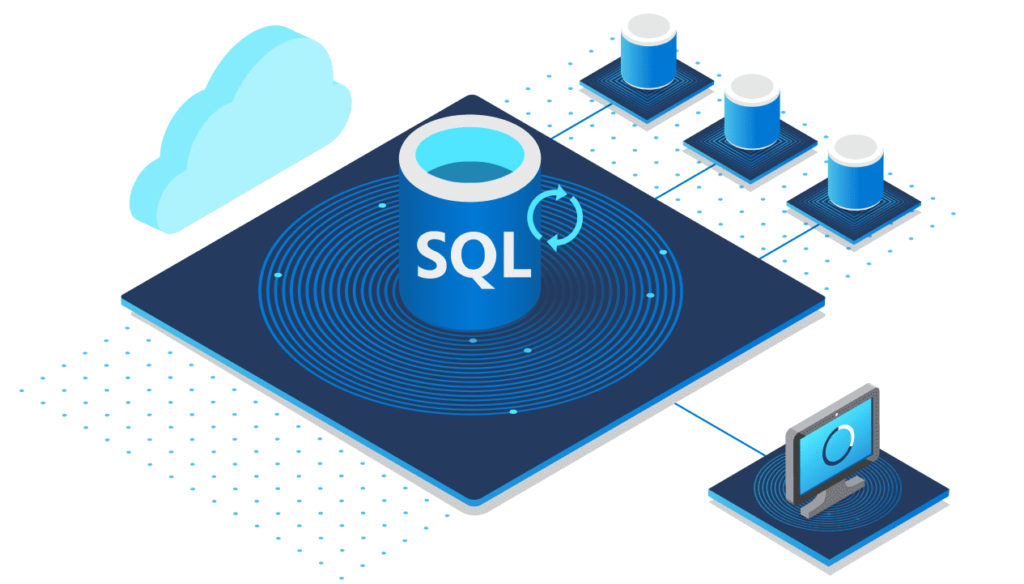
The complexity of Java and SQL can vary depending on the context in which they are used and the specific tasks being performed. However, in general, here’s how their complexity compares:
Java
Java is an object-oriented programming language, which means it focuses on objects and classes. This paradigm requires understanding concepts like classes, objects, inheritance, polymorphism, and encapsulation.
Java has a rich set of syntax and structure, including control flow statements (if, else, switch), loops (for, while, do-while), exception handling, and more.
Java manages memory automatically through garbage collection, but developers still need to understand concepts like heap memory, stack memory, object lifecycle, and memory leaks.
Java supports concurrent programming with features like threads, synchronization, locks, and concurrent collections, which can introduce complexities related to race conditions, deadlocks, and thread safety.
Java has a vast ecosystem of APIs and libraries for various purposes, such as GUI development (Swing, JavaFX), networking (java.net), database connectivity (JDBC), and more. Understanding and effectively using these APIs can add complexity.
SQL
SQL is a declarative language, meaning you specify what you want to achieve rather than how to achieve it. This can make SQL queries seem simpler to write, especially for basic operations like SELECT, INSERT, UPDATE, and DELETE.
SQL involves designing relational database schemas, understanding concepts like normalization, relationships (one-to-one, one-to-many, many-to-many), indexing, and optimization. While the basics are straightforward, designing efficient and scalable databases can be complex.
Writing efficient SQL queries requires understanding query execution plans, indexing strategies, and performance optimization techniques. While basic queries may seem simple, optimizing complex queries can be challenging.
SQL databases support transactions and ensure ACID (Atomicity, Consistency, Isolation, Durability) properties. Understanding transaction management, concurrency control, and isolation levels can add complexity.
Working with SQL often involves tasks like database installation, configuration, backup and recovery, security management, and performance tuning, which require knowledge beyond basic SQL queries.
Final Word
We’ve learned about SQL and Java, two different computer languages. SQL helps work with databases, like asking questions or changing information, while Java is used for making computer programs or websites.
When comparing the two, it’s important to think about how they’re used, how easy they are to learn, and what kinds of problems they help solve.
Everyone’s experience may be different, so it’s essential to consider what works best for you. Overall, both SQL and Java have their challenges, but with practice and patience, you can learn to use them effectively.